Chips can be used to represent small blocks of information. They are most commonly used either for contacts or for tags.
Chips Styles
You can also style it easily with materialize admin theme pre defined color set classes.
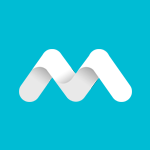
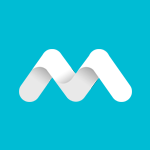
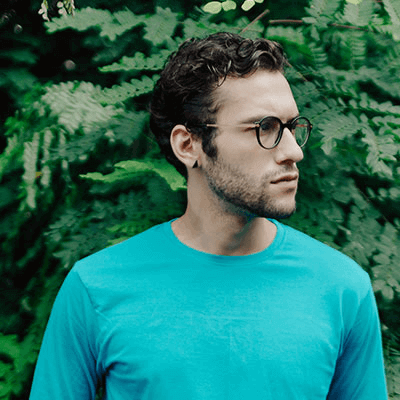
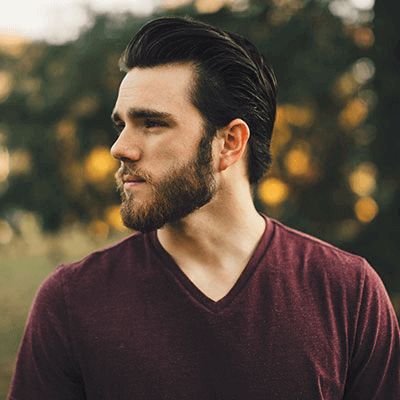
To add tags, just enter your tag text and press enter. You can delete them by clicking on the close icon or by using your delete button.
Set initial tags.
Use placeholders and override hint texts.
Use autocomplete with chips.
<!-- Default with no input (automatically generated) -->
<div class="chips"></div>
<div class="chips chips-initial"></div>
<div class="chips chips-placeholder"></div>
<div class="chips chips-autocomplete"></div>
<!-- Customizable input -->
<div class="chips">
<input class="custom-class">
</div>
document.addEventListener('DOMContentLoaded', function() {
var elems = document.querySelectorAll('.chips');
var instances = M.Chips.init(elems, options);
});
// Or with jQuery
$('.chips').chips();
$('.chips-initial').chips({
data: [{
tag: 'Apple',
}, {
tag: 'Microsoft',
}, {
tag: 'Google',
}],
});
$('.chips-placeholder').chips({
placeholder: 'Enter a tag',
secondaryPlaceholder: '+Tag',
});
$('.chips-autocomplete').chips({
autocompleteOptions: {
data: {
'Apple': null,
'Microsoft': null,
'Google': null
},
limit: Infinity,
minLength: 1
}
});
var chip = {
tag: 'chip content',
image: '', //optional
};
Options
Name | Type | Default | Description |
---|---|---|---|
data | Array | [] | Set the chip data (look at the Chip data object) |
placeholder | String | '' | Set first placeholder when there are no tags. |
secondaryPlaceholder | String | '' | Set second placeholder when adding additional tags. |
autocompleteOptions | Object | {} | Set autocomplete options. |
limit | Integer | Infinity | Set chips limit. |
onChipAdd | Function | null | Callback for chip add. |
onChipSelect | Function | null | Callback for chip select. |
onChipDelete | Function | null | Callback for chip delete. |
Methods
Use these methods to interact with chips.
Because jQuery is no longer a dependency, all the methods are called on the plugin instance. You can get the plugin instance like this:
var instance = M.Chips.getInstance(elem);
/* jQuery Method Calls
You can still use the old jQuery plugin method calls.
But you won't be able to access instance properties.
$('.chips').chips('methodName');
$('.chips').chips('methodName', paramName);
*/
.addChip();
Add chip to input.
Arguments
Chip: Chip data object.
instance.addChip({
tag: 'chip content',
image: '', // optional
});
.deleteChip();
Delete nth chip.
Arguments
Integer: Index of chip.
instance.deleteChip(3); // Delete 3rd chip.
.selectChip();
Select nth chip.
Arguments
Integer: Index of chip.
instance.selectChip(2); // Select 2nd chip
Properties
Name | Type | Description |
---|---|---|
el | Element | The DOM element the plugin was initialized with. |
options | Object | The options the instance was initialized with. |
chipsData | Array | Array of the current chips data. |
hasAutocomplete | Boolean | If the chips has autocomplete enabled. |
autocomplete | Object | Autocomplete instance, if any. |